How To: Use the Map Element in the Strategy Builder
We discussed examples of how to use the Generate and Enhance elements in a previous post. With the Map element, you can modify existing Recommendation fields and create new fields that can be passed to Flow as input variables. In this post, we’ll take a closer look at the Map element. Like Generate and Enhance, the output is always a list of recommendations, however, unlike the other two elements, Map doesn’t require you to write any Apex code and relies instead on expressions and formulas.
Use Case 1: Modify Existing Recommendation Fields with Expressions
Suppose you have a Recommendation with the Description, “Thank you for being a loyal customer. We truly appreciate your business!” Now, with the Map element, you can personalize this Description by appending the name of the contact. For example, “Lauren Boyle, Thank you for being a loyal customer. We truly appreciate your business!” You can use this concept to make a variety of easy enhancements using the full power of the Salesforce formula operators and functions.
To do so, first add a Load element to the canvas and load all of the recommendations you want to change (you could also choose to add a Generate element and pass in dynamically generated recommendations). Then, add the Map element to the canvas. In the Map Values to Fields section, set the Description field to be $Record.Contact.Name+ “, ” + Description.

Make sure the Map element (Personalized Thank You) is placed after the Load element. It will transform the Descriptions of all recommendations flowing into it.

When you execute the strategy, your recommendations will look like the image on the right and include the contact name for the current case.
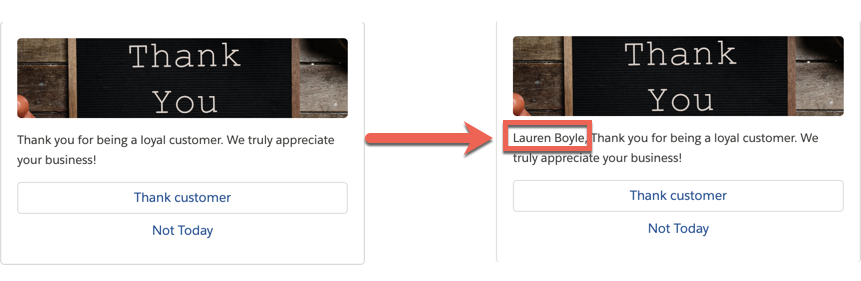
Use Case 2: Create New Fields That Can Be Passed as Inputs to Flow as Input Variables
The Einstein Next Best Action Lightning Component passes two default variables to all Flows – contextRecordId and recommendationId. In the Flow, you can do a Get Records and retrieve the current record on which the strategy is displayed (e.g. Case or Account) or the accepted recommendation. See this post for detailed instructions. With the Map element, you can create new “virtual” fields and pass them to the flow as input variables, in addition to the default ones. This scenario is most useful when you’re working with dynamically created recommendations and want to pass some context to the Flow. Bear in mind that in the case of dynamic recommendations, there is no recommendationId to pass to the Flow.
For example, let’s suppose you create a strategy that generates a set of accounts that you should connect with because the last contact was more than 90 days ago. Check out our Integration Guide for instructions on how to build such a strategy. When the recommendation is accepted, you want to launch a flow that references the selected account. Thus, you want to pass the Account Id to the Flow.

First, make a small tweak to the Recommendation object and add a text field called AccountId__c. Second, in the Apex action responsible for generating recommendations, set the AccountId__c with the ID of the generated account: AccountId__c = account.Id. The modified class would look like the following.
global class Generate_GetAccountsToFollowUp {
@InvocableMethod(label='Accounts to Follow Up Today'
description='Recommend accounts the current user should follow up on today')
global static List<List<Recommendation>> getAccounts(List<String> inputData){
List<List<Recommendation>> outputs = new List<List<Recommendation>>();
Integer daysSinceLastContact;
Account[] accounts = [SELECT Name, Description, LastContactDate__c, OwnerId FROM Account WHERE OwnerId = :inputData[0]];
List<Recommendation> recs = new List<Recommendation>();
for (Account account:accounts) {
if (account.LastContactDate__c != null){
daysSinceLastContact = account.LastContactDate__c.daysBetween(date.today());
if (daysSinceLastContact > 30){
Recommendation rec = new Recommendation(
Name = account.Name,
Description = 'Connect with the ' + account.Name + ' account, the last interaction was '+ daysSinceLastContact + ' days ago.',
ActionReference = 'accountOutreach',
AcceptanceLabel = 'View',
AccountId__c = account.Id
);
recs.add(rec);
}
}
}
outputs.add(recs);
return outputs;
}
}
Third, in the accountOutreach flow, create a text variable, called “accountId,” and mark it as “Available for input.”

Next, add a Map element after the Generate element.

Finally, in the Map element, create a text variable called accountId and set it to be AccountId__c. The variable name MUST match the name of the flow input variable.

The dynamically generated account Id will now be passed as an input variable to the Flow (accountId) and can be referenced to do things like Get Account details.

For example, send an email to each account.
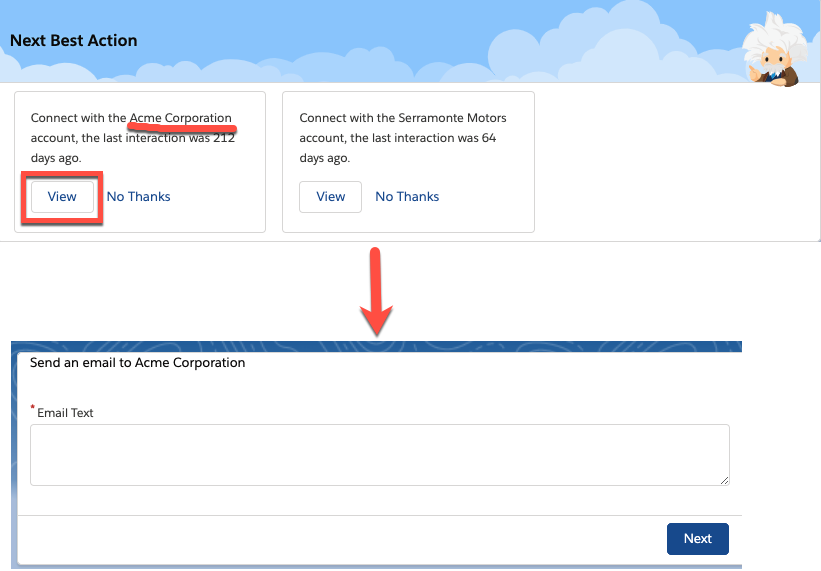